States
To create a State that can change between scripts according to a symbol you should use this scripts.
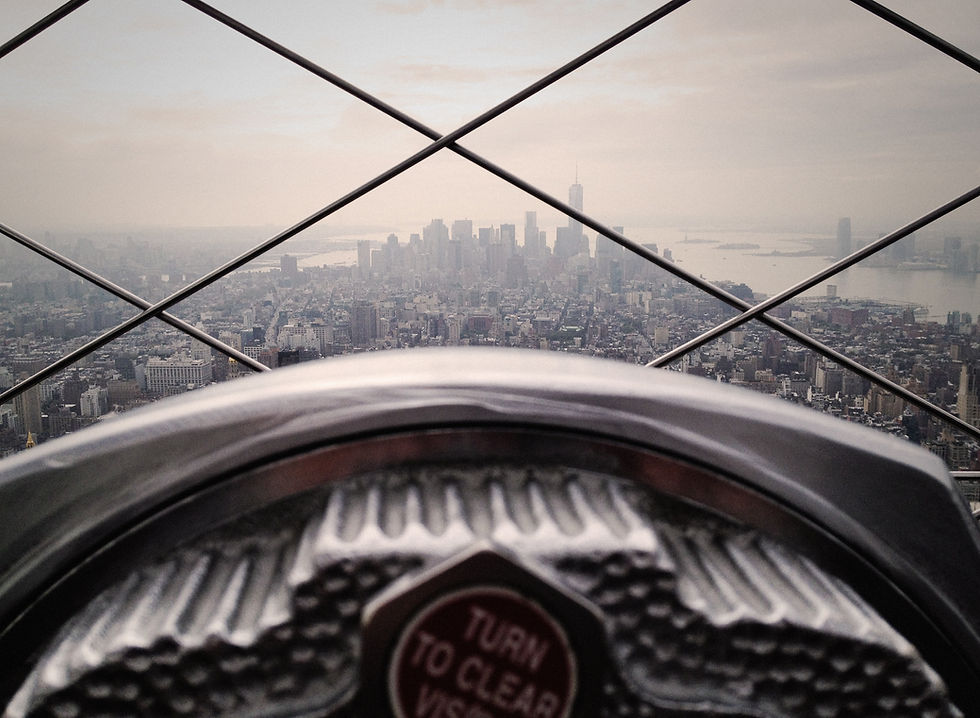
Script called Test:
using System.Collections; using System.Collections.Generic; using UnityEngine; using UnityEngine.SceneManagement; public class Test : MonoBehaviour { //Implementacion de un autómata private AState current; private ASymbol t, g, p, b; private MonoBehaviour currentBehaviour; void Start (){ AState sleeping = new AState ("sleeping", typeof(Sleeping)); AState eating = new AState ("eating", typeof(Eating)); AState peeing = new AState ("peeing", typeof(Peeing)); AState playing = new AState ("playing", typeof(Playing)); AState dead = new AState ("dead", typeof(Dead)); t = new ASymbol ("treat"); g = new ASymbol ("good boy"); p = new ASymbol ("pet"); b = new ASymbol ("bad boy"); sleeping.AddTransition (t, eating); sleeping.AddTransition (b, peeing); sleeping.AddTransition (p, playing); eating.AddTransition (g, peeing); peeing.AddTransition (b, dead); dead.AddTransition (g, sleeping); playing.AddTransition (b, dead); current = sleeping; currentBehaviour = gameObject.AddComponent (current.Behaviour) as MonoBehaviour; } void Update (){ //print (current.Name); if (Input.GetKeyUp (KeyCode.T)) { //current = current.ApplySymbol (t); ApplySymbol (t); } if (Input.GetKeyUp (KeyCode.G)) { //current = current.ApplySymbol (g); ApplySymbol (g); } if (Input.GetKeyUp (KeyCode.P)) { //current = current.ApplySymbol (p); ApplySymbol (p); } if (Input.GetKeyUp (KeyCode.B)) { //current = current.ApplySymbol (b); ApplySymbol (b); } if (Input.GetKeyUp (KeyCode.Return)) SceneManager.LoadScene ("Scene2"); } private void ApplySymbol(ASymbol symbol){ AState previous = current; current = current.ApplySymbol (symbol); if (previous != current) { //change script Destroy(currentBehaviour); currentBehaviour = gameObject.AddComponent (current.Behaviour) as MonoBehaviour; } } }
You'll need to add two other scripts called AState and ASymbol:
AState:
using System.Collections; using System.Collections.Generic; using UnityEngine; using System; public class AState{ private string name; private Type behaviour; //each of the states needs to know where to go Dictionary<ASymbol, AState> transition; //property //equivalent to get/set method public string Name { get { return name; } } public Type Behaviour{ get{ return behaviour; } } public AState(string name, Type behaviour){ this.name = name; this.behaviour = behaviour; transition = new Dictionary<ASymbol, AState> (); } public void AddTransition(ASymbol key, AState value){ transition.Add (key, value); } public AState ApplySymbol (ASymbol key){ if (transition.ContainsKey (key)) return transition [key]; //return null; return this; } }
ASymbol:
using System.Collections; using System.Collections.Generic; using UnityEngine; public class ASymbol { private string name; public string Name{ get{ return name; } } public ASymbol (string name){ this.name = name; } }
And add different state scripts like: Dead, Eating, Peeing, Playing and Sleeping.
They all have the same content:
using System.Collections; using System.Collections.Generic; using UnityEngine; public class Dead : MonoBehaviour { // Use this for initialization void Start () { } // Update is called once per frame void Update () { print ("It's Dead!"); } }