How to make random generated falling objects
- Oscar Acosta Mendoza
- Sep 11, 2017
- 2 min read
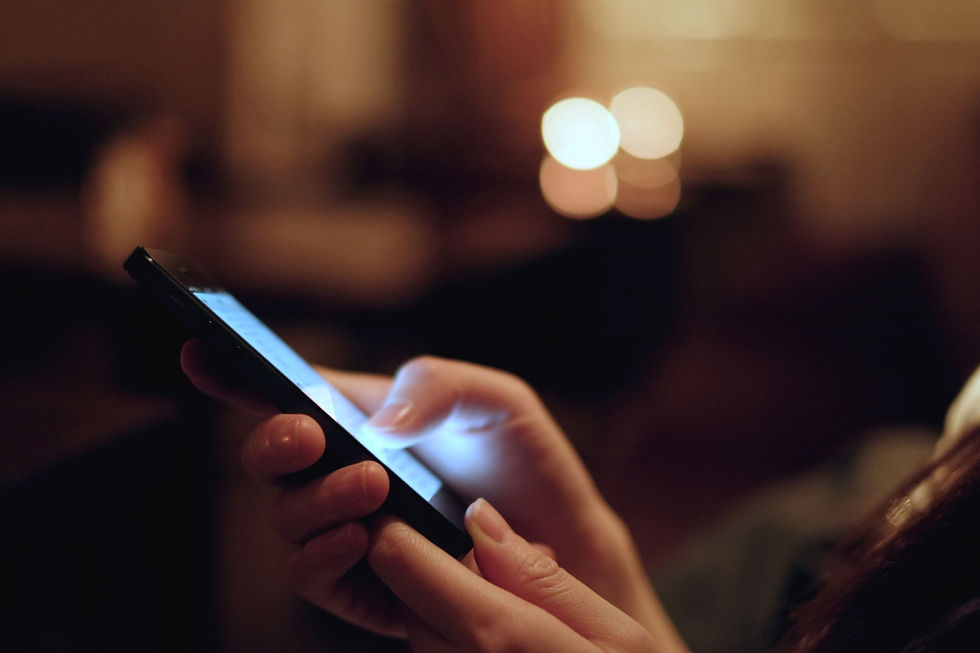
First we create a prefab box enemy with Rigidbody, and you go to the cannon script and paste this in the Update() method.
if ((int)Time.time % 3 == 0 && !enemy) { enemy = true; Vector3 randompos = new Vector3(Random.Range(-4,4),8,0); GameObject clone = Instantiate(boxes, randompos, transform.rotation) as GameObject; } else if(enemy && (int)Time.time % 3 != 0) { enemy = false; }
In this code we are checking on each frame if the time elapsed module 3 (seconds) is cero, this is to generate a clone of the enemy every three seconds in a random position.
to achieve the randomness of the position in X axis, we use the Random.Range(min,max) which returns a random number in the ranges you've specified.
Also we use a flag to prevent multiple enemy generation in the full second that the condition applies.
Full Code
using System.Collections; using System.Collections.Generic; using UnityEngine;
public class Cannon : MonoBehaviour { public float speedX; public float speedZ; //Vector used to restrain the rotation to Z axis only.
public Vector3 vectorZ = new Vector3(0f, 1f, 0f); public GameObject original; public GameObject boxes; public bool enemy = false;
// Use this for initialization void Start() { speedX = 5f; speedZ = 50f; }
// Update is called once per frame void Update() { //Horizontal Movement float h = Input.GetAxis("Horizontal"); transform.Translate(speedX * h * Time.deltaTime, 0, 0, Space.World);
//Rotation float v = Input.GetAxis("Vertical"); transform.Rotate(speedZ * vectorZ * v * Time.deltaTime, Space.Self);
if (Input.GetKeyUp(KeyCode.Space)) { //Cloning GameObject clone = Instantiate(original, //GameObject clone = Instantiate (Projectile.Instance, transform.position, transform.rotation) as GameObject; //make it at the same location that the canon Rigidbody rb2 = clone.GetComponent<Rigidbody>(); //retrieve a reference to someone else's components //Destroy (rb2); } print((int)Time.time); if ((int)Time.time % 3 == 0 && !enemy) { enemy = true; Vector3 randompos = new Vector3(Random.Range(-4,4),8,0); GameObject clone = Instantiate(boxes, randompos, transform.rotation) as GameObject; } else if(enemy && (int)Time.time % 3 != 0) { enemy = false; } } }
Finally we want the enemy boxes to destroy when a projectile hits them and when they hit the plane.
using System.Collections; using System.Collections.Generic; using UnityEngine;
public class Boxes : MonoBehaviour { // Use this for initialization void Start() {
}
// Update is called once per frame void Update() {
}
void OnCollisionEnter(Collision c) { if (c.transform.name == "Projectile(Clone)") { Destroy(c.gameObject); Destroy(this.gameObject); } if (c.transform.name == "Plane") { Destroy(this.gameObject); } //print (c.transform.name); } }
Comments